What’s a fundamental thing that is missing in the examples we’ve done so far? Hint: it’s pretty fundamental in games.
Answer: player interaction! So far, the player has done nothing but watch the slimes move, turn, etc. Let’s change that now. For example, let’s make the slime change color when it collides with something.
For that, we need to introduce a fundamental concept: messages.
A message is just a packet of information that gets sent from the engine to an actor, or from an actor to another actor. The message tells the actor that something happened, and gives the actor a chance to react to it. The code that reacts to a message is called the message handler function.
You have been using messages already! Guess what: Tick is a message, so when you write your onTick function, you are in fact handling the Tick message. The tick message gets delivered to every actor on every tick.
Another message that the engine delivers to actors automatically is the Collision message, which indicates that another actor has collided with it. Let’s try to write a handler for that message and see what happens.
Go back to your slime and edit My Block. Replace the code with this:
export function onCollision() { // Make it blue. setTint(0, 0, 1); }
We set things up so that when the slime hits another actor, it will set its color to blue.
Try it out. When you bump against the slime, it turns blue.
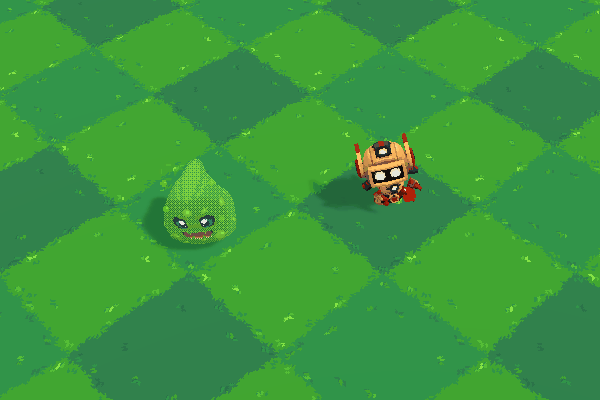
Great, right?