Memory means a set of values that an actor or a card remembers. You can store variables in memory so you can access them later. It also gets included in the save file, so whatever values you store are persisted if you save the game and load it again.
There are two levels of memory:
- Card memory: This is the memory that’s private to your card on this actor. It is not shared with other cards, and not shared with other actors. This is the recommended place to put most of your state, unless you need to share that state between cards.
- Actor memory: This is the memory that belongs to the actor as a whole, and is shared by all the cards that exist on the same actor. This is accessible through the mem global. Use this sparingly, since what you write here can overwrite what other cards have written.
Let’s use card memory to create a smoother gate. When the player bumps into it, we will store a variable in card memory called triggered (it could be called anything else, it’s just a variable!), and it will represent the fact that the gate has been triggered by the user.
Then, instead of opening the gate only while it’s being collided against, we will use the onTick function to slowly open it if the triggered variable is set to true.
To access the card’s memory, simply use the card object:
export function onTick() { if (card.triggered) { moveLeft(2); } } export function onCollision() { card.triggered = true; }
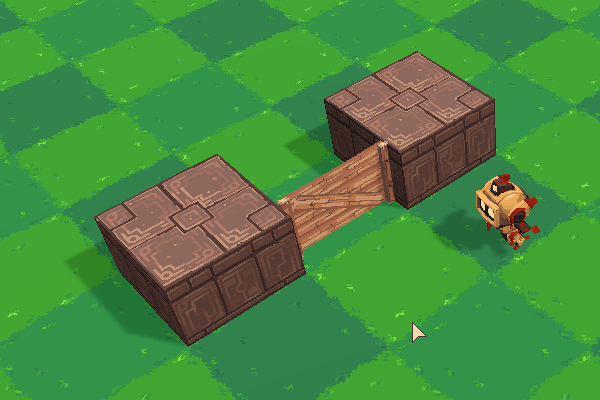
Much better, right? What is happening in this code is:
- onTick gets called every frame. But we don’t do anything because card.triggered hasn’t been set yet. So the gate stays closed.
- The player bumps into the gate.
- onCollision now gets called on the gate.
- We set card.triggered to true.
- onTick continues to get called every frame, but now that card.triggered is set, we call moveLeft(2) That function moves the actor to its left with a speed of 2 meters/second.