Now let’s do a more complicated example: a button that launches a rocketship.
There are 2 actors in this example: the rocketship and the button. Hitting the button will cause it to send a “Launch” message to the rocketship. The rocketship, upon receiving that message, will start to go up slowly.
So let’s build the scene first:
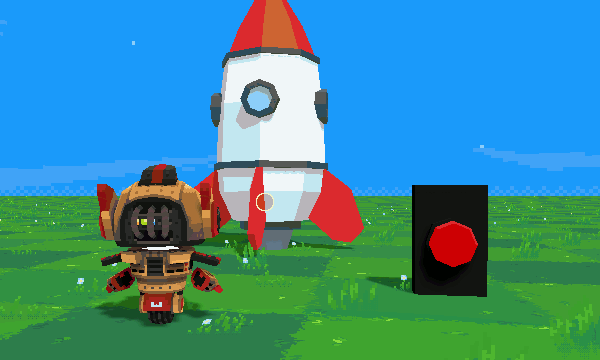
Nothing fancy. Ok, now let’s start with the code for the ship:
// Ship script export function onTick() { // If rocket was launched, move up at 2 units/second. if (card.launched) { moveUp(2); } } // This gets called when we receive the "Launch" message // (sent by the button). export function onLaunch() { card.launched = true; } export function onInit() { card.launched = false; }
Notice how the rocket is programmed to just sit there doing nothing until the card.launched variable is set to true, at which point on every tick it will call moveY to move up at 2 units/second.
When the rocket receives the Launch message (which the button will send, as we will show next), then it sets the card.launched variable to true, causing the motion to begin.
So here is what we write for the button:
// Declare the "rocket" property of this script, of type Actor: export const PROPS = [ propActor("rocket") ]; // This gets called when the player collides with the button. export function onCollision() { // Tell rocket to launch. send(props.rocket, "Launch"); }
There is a funny bit we haven’t seen before. This thing:
export const PROPS = [ propActor("rocket") ];
This indicates that this script has an actor property that can be set. This is similar to other properties we saw before (propNumber and propColor), but the value of this property is a reference to another actor.
To assign it, go to the block on the button, and click on the field. You can then select the rocket to set the value of that field, and from that point on you can refer to the rocket from your block as props.rocket.
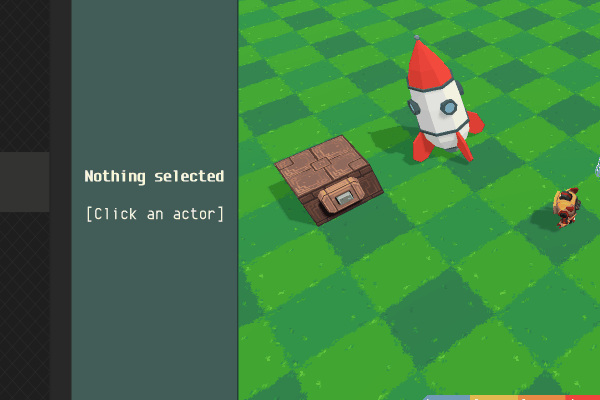
This is used in onCollision, in which we use the send function to send a message to the rocket. The message is simply Launch, which causes the rocket’s onLaunch function to be called, which itself causes the rocket to start going up.
Here is the result:
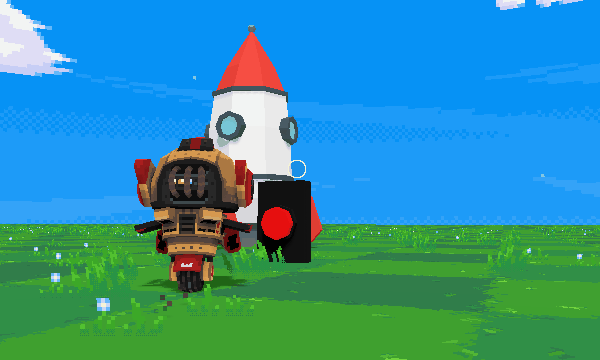